Nowadays most of the android devices
ship with a youtube player in it. In this post we will see how to
play a youtube video in android, provided the youtube player
application is already installed in your mobile.It is done by
creating an ACTION_VIEW Intent and starting the activity.This example
uses a standard youtube URL
So lets Start:
1.Create an android application project
with any projectname , an activity named MainActivity
and
a layout file named
activity_main
2.Now
design the UI activity_main.xml
for the MainActivity.java with a button to initiate the youtube video
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".MainActivity" > <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_marginTop="105dp" android:text="Play Youtube Video" /> </RelativeLayout>
3.Now create the MainActivity with ACTION_VIEW intent to start the youtube video on clicking the button
MainActivity.java:
import android.app.Activity; import android.content.Intent; import android.net.Uri; import android.os.Bundle; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; public class MainActivity extends Activity { Button b; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); b = (Button) findViewById(R.id.button1); b.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { // TODO Auto-generated method stub String video_path = "https://www.youtube.com/watch?v=qpQtlAW3mag"; Uri uri = Uri.parse(video_path); uri = Uri.parse("vnd.youtube:" + uri.getQueryParameter("v")); Intent intent = new Intent(Intent.ACTION_VIEW, uri); startActivity(intent); } }); } }4.Include internet permission <uses-permission android:name="android.permission.INTERNET"/> in your manifest file
5.Run
the application in a youtube installed android device the output
should be similar the the one as follows
Output:
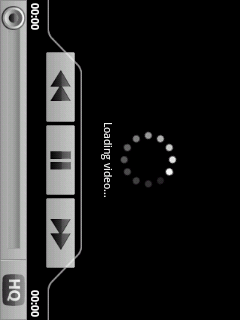