In this example we will demonstrate how
to implement sliding drawer in android. SlidingDrawer is a special
widget which allows user to drag some content into the screen from
outside and hide them if not needed. It has a handle and content ,
dragging the handle in shows the content and dragging the handle out
hides the content.
So lets start:
1.Create
a new project File
->New -> Project ->Android ->Android Application Project.
While creating the new project, name the activity as
SlidingDrawerActivity(SlidingDrawerActivity.java)
and layout as main.xml.
Press
Ctrl+Shift+O for missing imports after typing the code.
SlidingDrawerActivity.java
2.Now design the UI for the above activity in the activity_sliding_drawer.xml file .
public class SlidingDrawerActivity extends Activity implements OnClickListener { Button slideButton, b1, b2, b3; SlidingDrawer slidingDrawer; public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_sliding_drawer); slideButton = (Button) findViewById(R.id.slideButton); slidingDrawer = (SlidingDrawer) findViewById(R.id.SlidingDrawer); b1 = (Button) findViewById(R.id.Button01); b2 = (Button) findViewById(R.id.Button02); b3 = (Button) findViewById(R.id.Button03); b1.setOnClickListener(this); b2.setOnClickListener(this); b3.setOnClickListener(this); slidingDrawer.setOnDrawerOpenListener(new OnDrawerOpenListener() { @Override public void onDrawerOpened() { slideButton.setText("V"); } }); slidingDrawer.setOnDrawerCloseListener(new OnDrawerCloseListener() { @Override public void onDrawerClosed() { slideButton.setText("^"); } }); } @Override public void onClick(View v) { Button b = (Button) v; Toast.makeText(SlidingDrawerActivity.this, b.getText() + " is Clicked :)", Toast.LENGTH_SHORT).show(); } }
2.Now design the UI for the above activity in the activity_sliding_drawer.xml file .
activity_sliding_drawer.xml
3.Run the project by rightclicking project Run as → android project.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="fill_parent" android:layout_height="fill_parent" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" android:text="Drag the control at the bottom" android:textSize="20dp" tools:context=".SlidingDrawerActivity" /> <SlidingDrawer android:id="@+id/SlidingDrawer" android:layout_width="wrap_content" android:layout_height="250dip" android:layout_alignParentBottom="true" android:content="@+id/contentLayout" android:handle="@+id/slideButton" android:orientation="vertical" android:padding="10dip" > <Button android:id="@+id/slideButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="^" > </Button> <LinearLayout android:id="@+id/contentLayout" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="vertical" android:padding="10dip" > <Button android:id="@+id/Button01" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_margin="2dp" android:text="Button 1" > </Button> <Button android:id="@+id/Button02" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_margin="2dp" android:text="Button 2" > </Button> <Button android:id="@+id/Button03" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_margin="2dp" android:text="Button 3" > </Button> </LinearLayout> </SlidingDrawer> </RelativeLayout>
3.Run the project by rightclicking project Run as → android project.
Output:
The
output of this example would be similar to the one as follows
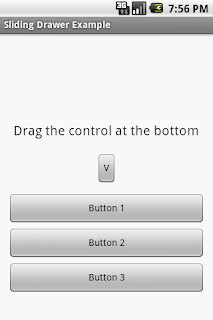