In this sample application we will put
the android accelerometer sensor into use . Nowadays accelerometer is
used more in motion games where the user tilts the phone for a
specific action to take place or used for shake detection. It has
become common to shake the device to reset the application or erase a
canvas in paint apps.
In this example we will change the
image in the ImageView with respect to the users actions (like
tilting the phone up,down right and left).Like we used for the
proximity sensor example we use the SensorManager
class and get the accelerometer sensor service. On the overridden
method onSensorChanged
we
monitor the sensor values of all 3 axises (x ,y & z). Keep a
threshold valued of 2.0 for center and change the image according the
the axis values. Hope you got the idea.
Now
lets start:
1.Create
a new project File
->New -> Project ->Android ->Android Application Project.
While creating the new project, name the activity as
AccelerometerActivity(AccelerometerActivity.java)
and layout as activity_accelerometer.xml.
2.Now
let us design the UI for the AccelerometerActivity i.e
activity_accelerometer.xml
with an ImageView and a TextView.Have images in drawable folder to
differentiate each tilting motion(Here I have images for
center,top,right,bottom and left).
activity_accelerometer.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/relative" android:layout_width="fill_parent" android:layout_height="fill_parent" > <TextView android:id="@+id/txt" android:layout_width="fill_parent" android:layout_height="wrap_content" android:textSize="20dp" android:text="Tilt the phone to see the difference" /> <ImageView android:id="@+id/imageView1" android:layout_width="fill_parent" android:layout_height="fill_parent" android:layout_below="@+id/txt" android:layout_centerHorizontal="true" android:scaleType="fitXY" android:src="@drawable/center" /> </RelativeLayout>
3.Now
in the AccelerometerActivity use a logic to identify the tilting
action and replace the images accordingly. Do not forget to
unregister the sensor to save battery.
AccelerometerActivity.java
import android.app.Activity; import android.content.Context; import android.hardware.Sensor; import android.hardware.SensorEvent; import android.hardware.SensorEventListener; import android.hardware.SensorManager; import android.os.Bundle; import android.widget.ImageView; import android.widget.TextView; public class AccelerometerActivity extends Activity implements SensorEventListener { private SensorManager mSensorManager; private Sensor mAccelerometer; TextView title; ImageView iv; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_accelerometer); mSensorManager = (SensorManager) getSystemService(Context.SENSOR_SERVICE); mAccelerometer = mSensorManager .getDefaultSensor(Sensor.TYPE_ACCELEROMETER); title = (TextView) findViewById(R.id.txt); iv = (ImageView) findViewById(R.id.imageView1); } @Override public void onAccuracyChanged(Sensor arg0, int arg1) { // TODO Auto-generated method stub } @Override public void onSensorChanged(SensorEvent event) { float x = event.values[0]; float y = event.values[1]; float z = event.values[2]; if (Math.abs(x) > Math.abs(y)) { if (x < 0) { iv.setImageResource(R.drawable.right); } if (x > 0) { iv.setImageResource(R.drawable.left); } } else { if (y < 0) { iv.setImageResource(R.drawable.top); } if (y > 0) { iv.setImageResource(R.drawable.bottom); } } if (x > (-2) && x < (2) && y > (-2) && y < (2)) { iv.setImageResource(R.drawable.center); } } @Override protected void onResume() { super.onResume(); mSensorManager.registerListener(this, mAccelerometer, SensorManager.SENSOR_DELAY_NORMAL); } @Override protected void onPause() { super.onPause(); mSensorManager.unregisterListener(this); } }
4.Run
the project by rightclicking project Run
as → android project in
an android device.
Output:
The
output of this example would be similar to the one as follows:
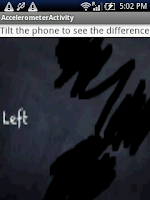
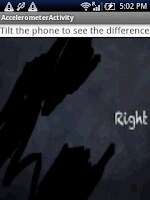